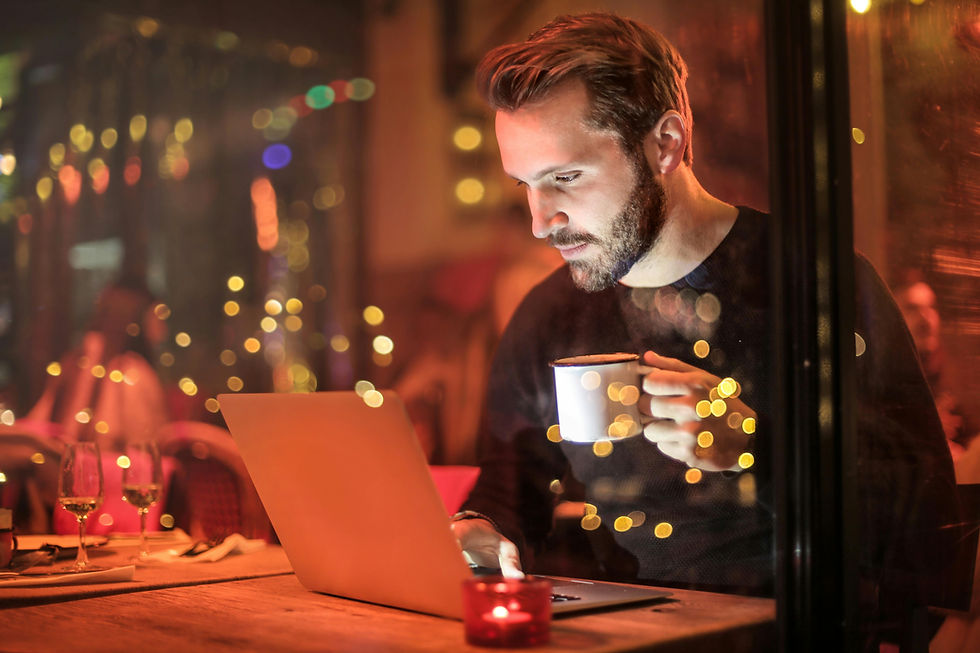
SQL injection is a type of cyber attack where an attacker can execute malicious SQL statements in a web application's database. This can lead to unauthorized access, data manipulation, and other security breaches. To prevent SQL injection, you can follow several best practices:
1. Use Parameterized Statements (Prepared Statements):
- Instead of dynamically building SQL queries by concatenating strings, use parameterized statements provided by your database or programming language. Parameters are placeholders that are later filled with user input.
Example (in Python using SQLite):
```python
cursor.execute("SELECT * FROM users WHERE username = ? AND password = ?", (user_input_username, user_input_password))
```
2. Input Validation:
- Validate and sanitize user input before using it in SQL queries. Ensure that input adheres to expected formats and ranges. Use whitelists to accept only known good values.
Example (in PHP):
```php
$username = mysqli_real_escape_string($conn, $_POST['username']);
$password = mysqli_real_escape_string($conn, $_POST['password']);
```
3. Stored Procedures:
- Use stored procedures to encapsulate database logic. This can help prevent direct execution of arbitrary SQL code.
4. Least Privilege Principle:
- Ensure that the database user account used by your application has the minimum necessary permissions. Avoid using accounts with excessive privileges.
5. Database Firewalls:
- Implement database firewalls to monitor and filter SQL traffic, blocking suspicious or potentially harmful queries.
6. Regular Updates and Patching:
- Keep your database management system (DBMS) and application framework up to date with the latest security patches to address vulnerabilities.
7. Error Handling:
- Provide custom error messages to users without revealing sensitive information. Log detailed error messages internally for debugging purposes.
8. Web Application Firewall (WAF):
- Employ a WAF to filter and monitor HTTP traffic between a web application and the internet. It can help detect and block SQL injection attempts.
9. Security Audits and Code Reviews:
- Regularly conduct security audits and code reviews to identify and fix potential vulnerabilities in your application code.
10. Use an ORM (Object-Relational Mapping):
- If applicable, consider using an ORM framework that abstracts the database layer and automatically generates secure SQL queries.
Remember that a combination of these practices is often more effective than relying on just one. Regularly testing your application for vulnerabilities is crucial to maintaining a secure system.
Comments